How Can We Convert Base64 to PDF? A Step-by-Step Guide
In the digital world, converting Base64 encoded data to PDF is a common task that can streamline data handling and presentation. This article provides a comprehensive guide on how to convert Base64 to PDF using various programming languages, ensuring that your data is accurately transformed into a PDF format.
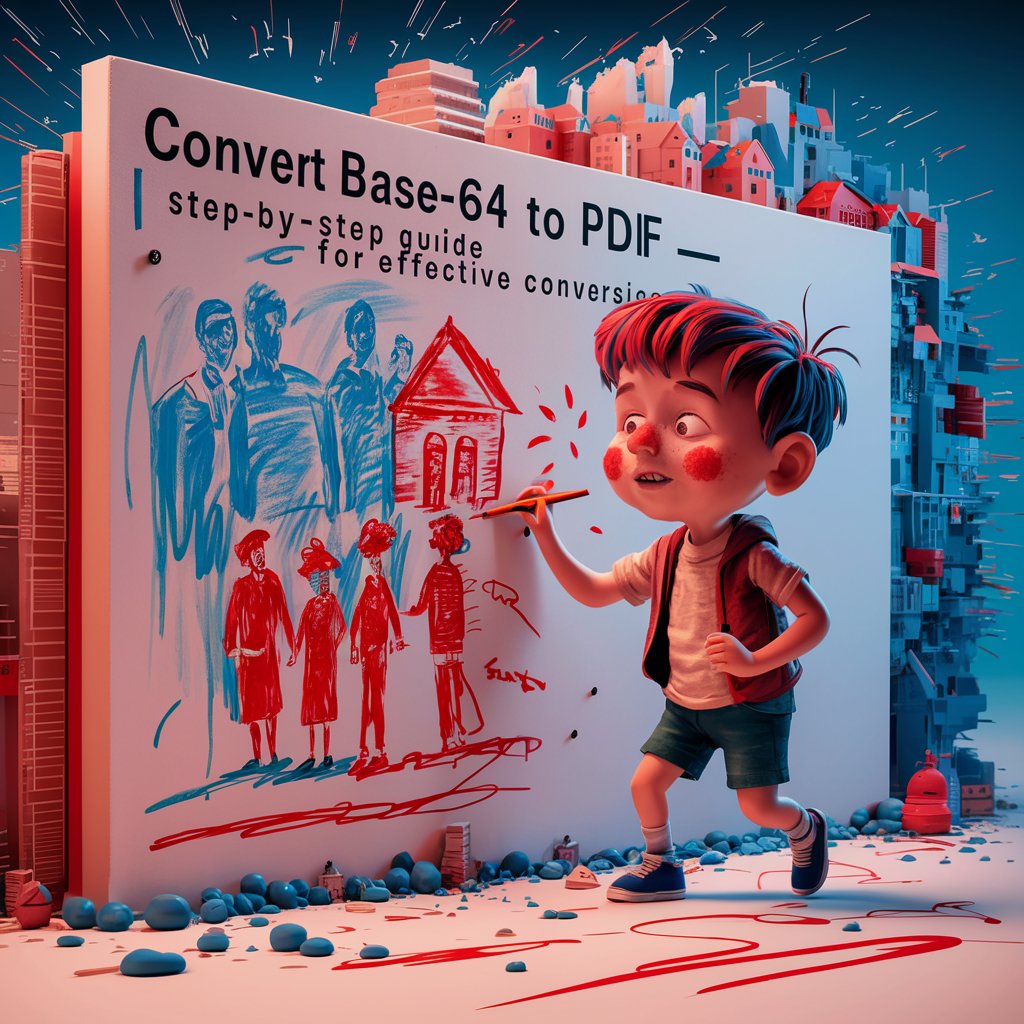
Introduction to Base64 and PDF
Base64 encoding is a method to convert binary data into a text format using ASCII characters. This is particularly useful for transmitting data over media that are designed to handle text. PDFs, on the other hand, are widely used for presenting documents in a manner independent of application software, hardware, and operating systems.
Why Convert Base64 to PDF?
Converting Base64 encoded data to PDF is useful for several reasons:
- Data Integrity: Ensures that binary data such as images and documents are preserved accurately.
- Ease of Sharing: PDF files are universally recognized and can be easily shared across different platforms.
- Professional Presentation: PDF format provides a clean and professional way to present data.
Methods to Convert Base64 to PDF
There are several ways to convert Base64 encoded data to PDF. Below are step-by-step guides using different programming languages.
Using Python
Python offers several libraries that can help convert Base64 to PDF. One popular library is PyPDF2
.
Step-by-Step Guide
- Install Required Libraries:
pip install PyPDF2
- Convert Base64 to PDF:
import base64
from PyPDF2 import PdfFileWriter, PdfFileReader
from io import BytesIO
# Base64 encoded PDF string
base64_string = "JVBERi0xLjcKJcfsj6IKNSAwIG9iaiA8PC9MZW5ndGg..."
# Decode Base64 to binary
pdf_bytes = base64.b64decode(base64_string)
# Write binary data to PDF
with open("output.pdf", "wb") as pdf_file:
pdf_file.write(pdf_bytes)
print("PDF file created successfully.")
Using JavaScript
JavaScript, particularly in a browser environment, can handle Base64 to PDF conversion effectively using Blob and FileReader APIs.
Step-by-Step Guide
- Create HTML and JavaScript:
<!DOCTYPE html>
<html>
<head>
<title>Base64 to PDF</title>
</head>
<body>
<button onclick="convertBase64ToPDF()">Convert Base64 to PDF</button>
<script>
function convertBase64ToPDF() {
// Base64 encoded PDF string
var base64String = "JVBERi0xLjcKJcfsj6IKNSAwIG9iaiA8PC9MZW5ndGg...";
// Decode Base64 string
var binaryString = atob(base64String);
var len = binaryString.length;
var bytes = new Uint8Array(len);
for (var i = 0; i < len; i++) {
bytes[i] = binaryString.charCodeAt(i);
}
// Create Blob and generate URL
var blob = new Blob([bytes], { type: "application/pdf" });
var url = URL.createObjectURL(blob);
// Create and click a link to download the PDF
var a = document.createElement("a");
a.href = url;
a.download = "output.pdf";
a.click();
URL.revokeObjectURL(url);
}
</script>
</body>
</html>
Using Node.js
Node.js can also handle Base64 to PDF conversion using the fs
(file system) module.
Step-by-Step Guide
- Install Node.js (if not already installed):
sudo apt-get install nodejs
- Create JavaScript File:
const fs = require('fs');
// Base64 encoded PDF string
const base64String = "JVBERi0xLjcKJcfsj6IKNSAwIG9iaiA8PC9MZW5ndGg...";
// Decode Base64 to binary
const pdfBuffer = Buffer.from(base64String, 'base64');
// Write binary data to PDF
fs.writeFile('output.pdf', pdfBuffer, (err) => {
if (err) throw err;
console.log('PDF file created successfully.');
});
Using Java
Java provides robust libraries for file handling, and Base64 encoding is supported through the
java.util.Base64
class.
Step-by-Step Guide
- Convert Base64 to PDF in Java:
import java.io.FileOutputStream;
import java.util.Base64;
public class Base64ToPDF {
public static void main(String[] args) {
// Base64 encoded PDF string
String base64String = "JVBERi0xLjcKJcfsj6IKNSAwIG9iaiA8PC9MZW5ndGg...";
try {
// Decode Base64 string to byte array
byte[] pdfBytes = Base64.getDecoder().decode(base64String);
// Write byte array to PDF file
FileOutputStream fos = new FileOutputStream("output.pdf");
fos.write(pdfBytes);
fos.close();
System.out.println("PDF file created successfully.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Conclusion
Converting Base64 encoded data to PDF is a straightforward process that can be accomplished using various programming languages. Whether you are working with Python, JavaScript, Node.js, or Java, the key steps involve decoding the Base64 string to binary data and then writing this data to a PDF file. By following the provided guides, you can ensure accurate and efficient conversion for your data needs.
FAQs
What is Base64 encoding? Base64 encoding is a method of converting binary data into a text format using 64 ASCII characters, ensuring data can be safely transmitted over text-based systems.
Why convert Base64 to PDF? Converting Base64 to PDF ensures data integrity, ease of sharing, and professional presentation of binary data.
Can Base64 encode any type of data? Yes, Base64 can encode any type of binary data, including images, documents, and multimedia files.
What tools are needed to convert Base64 to PDF? You can use various programming languages and their respective libraries, such as Python, JavaScript, Node.js, and Java.
Is the conversion process the same for all programming languages? The core concept of decoding Base64 and writing binary data to a PDF file is the same, but the implementation details vary across different programming languages.
Are there online tools for Base64 to PDF conversion? Yes, several online tools can perform Base64 to PDF conversion, but using programming languages offers more control and flexibility for automated workflows.
Inbound and Outbound Links Suggestions
Inbound Links:
- Understanding Data Encoding Techniques
- Best Practices for Data Conversion
- Enhancing Data Presentation with PDF
Outbound Links:
By mastering the process of converting Base64 to PDF, you can enhance your data handling capabilities, ensuring that your data is preserved and presented in the best possible manner.